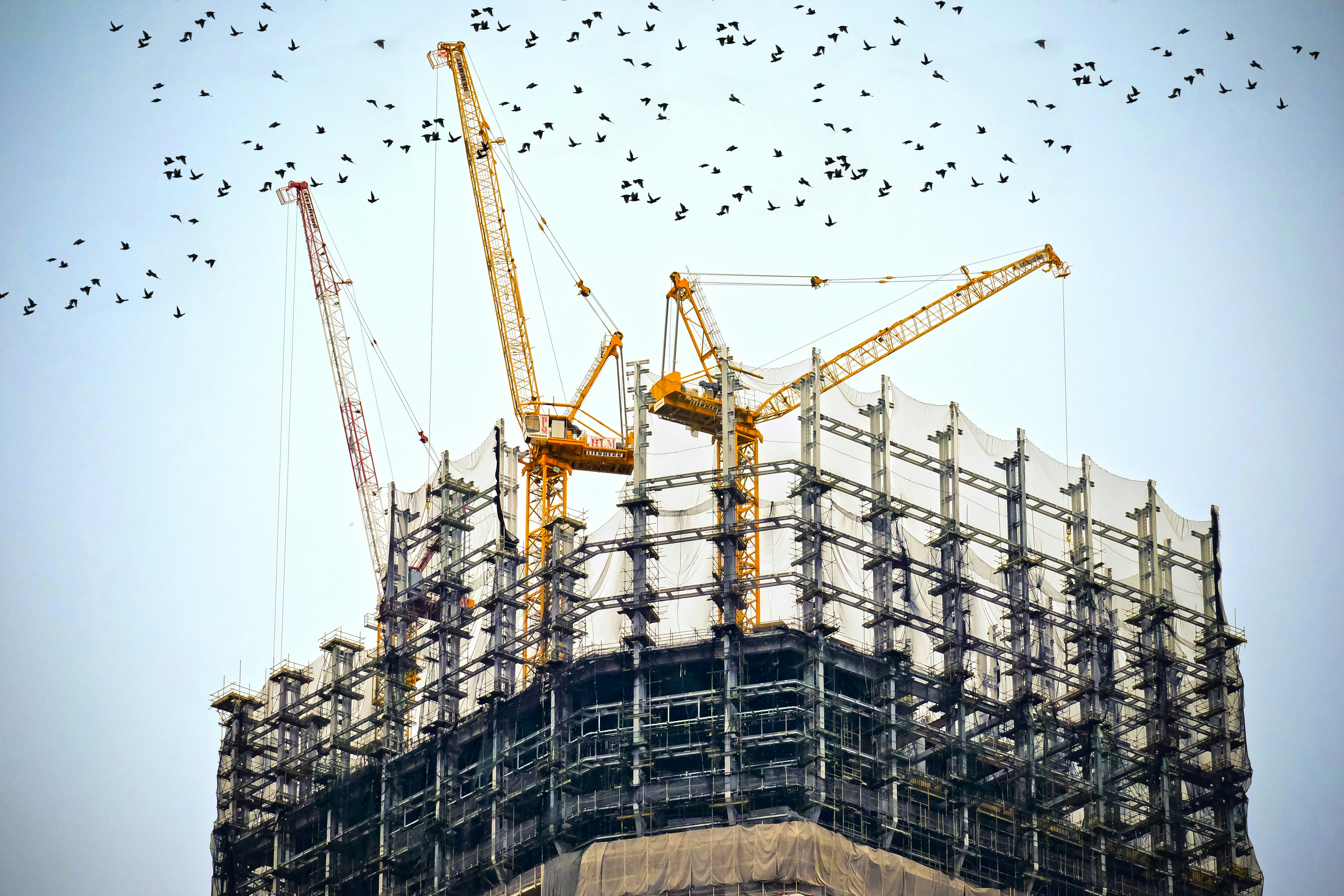
Introduction
Build systems help transform source code into executable programs. GNU Make is perhaps the most well-known build system, but CMake is gaining significant traction. This article aims to provide an introduction to CMake for developers who are already familiar with GNU Make. By the end of this guide, we hope you’ll have a more clear understanding of how to use CMake in your existing projects.
Syntax
GNU Make and CMake present starkly different approaches to build system syntax. GNU Make’s approach is similar to a traditional scripting style, requiring explicit rules for each target. This approach offers fine-grained control but can become verbose and repetitive for complex projects.
For example, in GNU Make, you might write:
%.o: %.c
$(CC) -c $(CFLAGS) $< -o $@
CMake, conversely, adopts a higher-level, declarative approach. Instead of specifying detailed rules, you describe your desired outcome, allowing CMake to determine the optimal method to achieve it.
In CMake, you might write:
add_executable(my_program main.c)
This abstraction can significantly reduce the amount of boilerplate code, especially for larger projects. However, it does require a shift in thinking from procedural to declarative programming. However, many developers find that CMake’s approach leads to more maintainable and less error-prone build configurations in the long run.
Cross-Platform Compatibility
One of CMake’s most compelling features is its robust cross-platform compatibility. While GNU Make excels in Unix-like environments, it can struggle with seamless integration on other platforms, particularly Windows.
CMake was designed from the ground up to be platform-agnostic. It acts as an abstraction layer, generating native build files for the target platform. This means you can write a single set of CMake instructions and generate appropriate build files whether you’re on Linux (Makefiles), Windows (Visual Studio projects), or macOS (Xcode projects).
For instance, this simple CMake script:
project(MyProject)
add_executable(MyProject main.cpp)
will generate the appropriate build system for your environment without additional configuration. This feature is particularly valuable for projects that need to support multiple platforms or for teams working in heterogeneous environments.
Out-of-Source Builds
CMake’s support for out-of-source builds is another significant advantage over GNU Make. While it’s possible to set up out-of-source builds with GNU Make, it requires manual configuration and can be error-prone.
CMake, on the other hand, treats out-of-source builds as the default behavior. This approach keeps your source directory clean and allows for multiple build configurations (e.g., debug and release) from the same source tree.
To create an out-of-source build with CMake:
mkdir build
cd build
cmake ..
This creates a separate build directory, placing all generated files and build artifacts there, leaving the source directory untouched. This separation not only keeps your project organized but also simplifies cleanup and allows for easy switching between different build configurations.
Configuration Files
GNU Make typically relies on a single Makefile
to manage build rules. While this approach works well for small projects, it can lead to unwieldy, monolithic configuration files as projects grow in complexity.
CMake encourages a modular approach to configuration. Instead of a single large file, CMake projects typically use multiple CMakeLists.txt
files distributed throughout the project hierarchy. Each CMakeLists.txt
file is responsible for its own directory and subdirectories.
For example:
# Root CMakeLists.txt
cmake_minimum_required(VERSION 3.10)
project(MyProject)
add_subdirectory(src)
add_subdirectory(tests)
# src/CMakeLists.txt
add_library(my_lib my_lib.cpp)
# tests/CMakeLists.txt
add_executable(test_my_lib test_my_lib.cpp)
target_link_libraries(test_my_lib my_lib)
This modular structure improves maintainability and makes it easier to understand the build configuration for each part of your project. It also allows for better encapsulation, as each subdirectory can have its own build settings without affecting the rest of the project.
Testing Support
Integrated testing support is another area where CMake shines. While GNU Make doesn’t provide built-in testing capabilities, requiring integration with external tools or custom Makefile rules, CMake offers CTest, a built-in testing framework.
With CTest, adding and running tests becomes straightforward:
enable_testing()
add_executable(my_test my_test.cpp)
add_test(NAME MyTest COMMAND my_test)
You can then run tests using the ctest
command:
cmake ..
make
ctest
CTest supports features like test fixtures, labels, and parallel execution. While it may not be as feature-rich as dedicated testing frameworks like Google Test or Catch2, it provides a solid foundation for basic testing needs and integrates seamlessly with the CMake ecosystem.
For more advanced testing requirements, CMake can easily integrate with external testing frameworks, providing flexibility to suit various project needs.
Integration with Other Tools
In modern software development, the ability to integrate smoothly with other tools in the development ecosystem is crucial. GNU Make, being a lower-level tool, often requires custom scripts or rules to integrate with other tools.
CMake, however, provides built-in modules for many common tasks and integrations. These include:
find_package()
for locating and using external librariescheck_cxx_compiler_flag()
for checking compiler feature support- Modules for generating documentation with Doxygen
- Support for building SWIG interfaces
Moreover, CMake has excellent integration with modern IDEs like CLion, Visual Studio Code, and Visual Studio. These IDEs can directly load and understand CMake projects, providing features like code navigation, auto-completion, and debugging out of the box.
This level of integration positions CMake not just as a build system, but as a comprehensive project management tool that can streamline the entire development workflow.
Ease of Use
Ease of use is subjective and often depends on individual experience and project requirements. GNU Make, with its long history and widespread use, has well-established patterns and extensive documentation. For developers already familiar with Make, writing Makefiles can feel intuitive and straightforward.
CMake, while offering powerful features, does have a steeper learning curve. Its syntax and concepts can feel unfamiliar to developers accustomed to the explicit rules of Makefiles. However, once past the initial learning phase, many developers find that CMake’s high-level abstractions and built-in commands for common tasks lead to more efficient and maintainable build configurations, especially for larger and more complex projects.
CMake’s ease of use shines in scenarios involving:
- Cross-platform development
- Projects with complex dependency structures
- Codebases that require frequent configuration changes
While the transition from GNU Make to CMake requires an initial investment in learning, the long-term benefits in terms of flexibility, maintainability, and integration capabilities often outweigh the upfront costs.
Conclusion
The journey from GNU Make to CMake represents more than just a change in build systems; it’s a shift in how we approach the entire build process. CMake’s high-level syntax, robust cross-platform support, out-of-source builds, modular configuration files, integrated testing capabilities, and extensive tool integrations offer compelling advantages, especially for larger and more complex projects.
However, the decision to transition should be based on your specific project needs, team expertise, and long-term goals. GNU Make remains a powerful and viable option, particularly for smaller projects or those deeply embedded in Unix-like environments.
Ultimately, both GNU Make and CMake are tools designed to facilitate the software development process. The best choice depends on your project’s specific requirements, your team’s expertise, and your development workflow. Whichever path you choose, the goal remains the same: to create efficient, maintainable, and high-quality software.