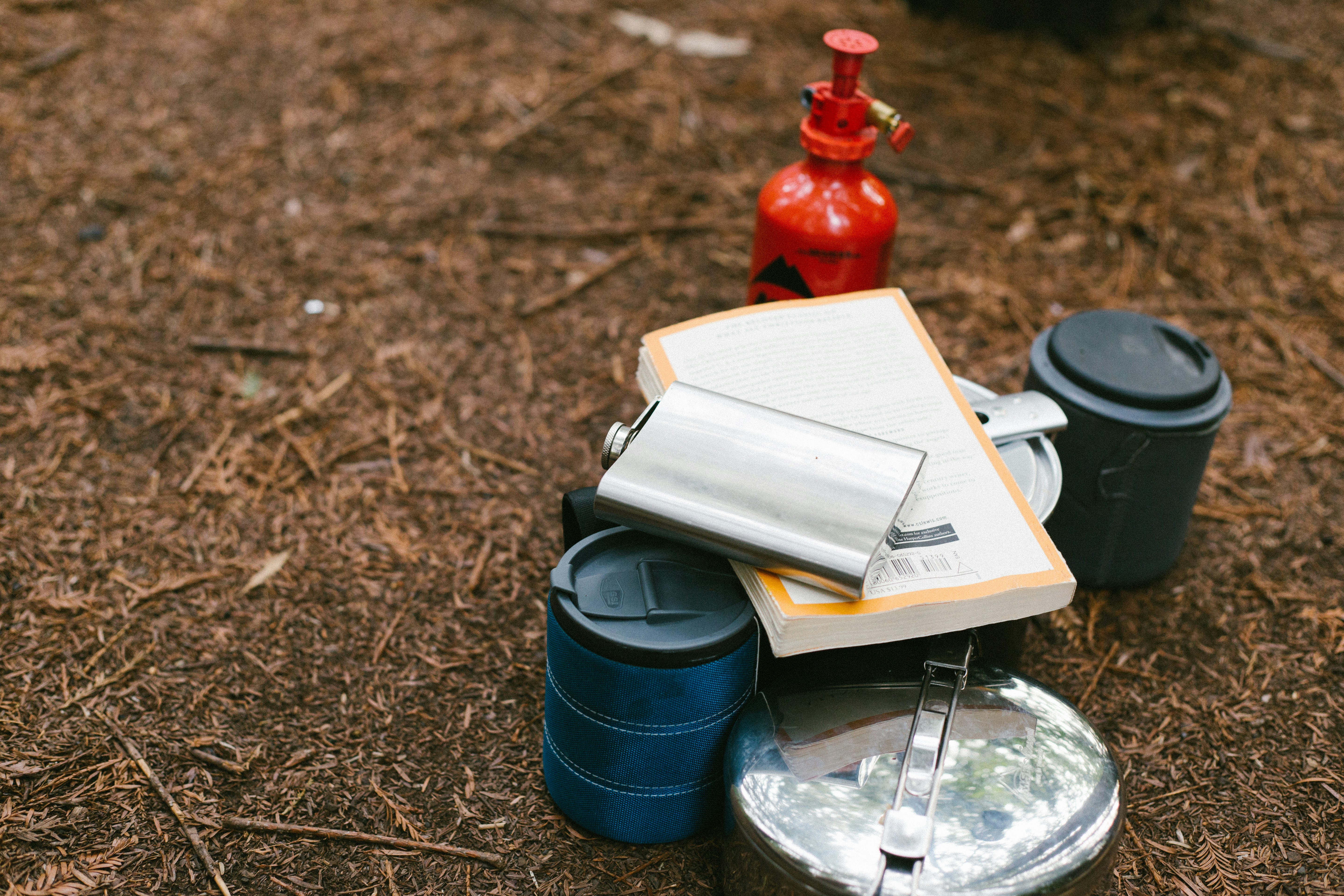
Flask is one of those frameworks that wins over developers by doing less. It’s lightweight, flexible, and doesn’t box you in with assumptions about how your application should work. But that same minimalism can also leave developers reaching for essential tools. That’s where Flask extensions come in. They fill in the blanks, providing powerful, pre-built solutions for everything from database handling to authentication, caching, and form validation.
Flask extensions are like building blocks that snap together perfectly with Flask’s core. You get the flexibility of a micro-framework but with the power to add only what you need—nothing more, nothing less. This modularity is key for developers managing complex applications or those looking to scale without adding unnecessary bloat. Instead of writing custom code for common tasks like user sessions or email integration, these extensions handle the heavy lifting and save you hours of work.
The right set of Flask extensions can make all the difference in your development workflow, helping you focus more on what makes your app unique and less on repetitive boilerplate. In this article, we’ll explore nine must-have Flask extensions that can turn your barebones app into a robust, feature-rich application—without sacrificing Flask’s signature simplicity.
1. Flask-SQLAlchemy
Flask-SQLAlchemy is one of the most widely-used extensions for Flask, and it’s easy to see why. At its core, Flask-SQLAlchemy integrates SQLAlchemy—an Object Relational Mapper (ORM)—with Flask, making database management smoother and more Pythonic. SQLAlchemy is highly regarded for its flexibility, allowing developers to interact with databases using Python objects without needing to write raw SQL. Flask-SQLAlchemy builds on this by adding convenient features for Flask users, simplifying setup and enabling seamless interaction with databases like PostgreSQL, MySQL, and SQLite.
Key Features and Strengths
-
ORM for Mapping Python Objects to Database Tables: Flask-SQLAlchemy simplifies database work by allowing developers to map Python objects directly to database tables, letting you interact with your database like any other Python object.
-
Supports Multiple Databases: Whether you’re using PostgreSQL, MySQL, or SQLite, Flask-SQLAlchemy can handle them all. This flexibility makes it suitable for projects of all sizes.
-
Simplifies Database Migrations: Paired with Flask-Migrate (which we’ll cover later), it helps you manage database schema changes without manual SQL.
Instructions
First, you’ll need to install the extension with pip:
pip install flask-sqlalchemy
After installation, it’s easy to configure Flask-SQLAlchemy in your application. First, define the database URI in your app’s configuration. For example, to use a PostgreSQL database:
app.config['SQLALCHEMY_DATABASE_URI'] = 'postgresql://username:password@localhost/yourdatabase'
Creating a Database Model:
Once configured, define your models by subclassing db.Model
:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
Flask-SQLAlchemy works well with Flask-Migrate to handle database migrations. Once you’ve defined your models, you can create and apply migrations effortlessly using Flask-Migrate’s CLI commands.
2. Flask-WTF
[Flask-WTF][] simplifies form creation and validation by integrating Flask with WTForms, a Python library designed for working with forms. If you’ve ever had to manually manage form fields and validation, Flask-WTF will save you a lot of time. It’s designed to be secure out of the box with CSRF protection and supports more complex use cases like file uploads and custom validators.
Key Features and Strengths
- Built-in CSRF protection.
- Easy form field creation and validation.
- Supports file uploads and custom validators.
Instructions
First, install Flask-WTF using pip:
pip install flask-wtf
Next, configure CSRF protection by setting a secret key in your Flask app:
app.config['SECRET_KEY'] = 'a_secure_random_key'
Now, you can define a form using WTForms. Here’s an example of a simple login form:
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField
from wtforms.validators import DataRequired
class LoginForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
password = PasswordField('Password', validators=[DataRequired()])
submit = SubmitField('Log In')
To render the form in a template, you can use Jinja2. In your
login.html
template, include the following:
<form method="POST" action="/login">
{{ form.hidden_tag() }}
{{ form.username.label }} {{ form.username() }}
{{ form.password.label }} {{ form.password() }}
{{ form.submit() }}
</form>
Flask-WTF takes care of the complexity of form handling, so you can focus on building the core features of your application.
3. Flask-Login
Flask-Login is a crucial extension for handling user authentication and session management in Flask applications. It’s designed to make implementing user logins, logouts, and session handling straightforward while giving you the flexibility to customize authentication logic. Whether you’re building a simple login system or a more complex user management feature, Flask-Login simplifies the heavy lifting.
Key Features and Strengths
- Simple login and logout functionality with minimal setup.
- Supports persistent user sessions, allowing users to remain logged in across requests.
- Easily integrates with custom user models, so you can define how users are stored and authenticated in your system.
Instructions
First, install Flask-Login using pip:
pip install flask-login
After installing, you need to integrate Flask-Login into your application. Start by importing the extension and initializing it:
from flask_login import LoginManager
login_manager = LoginManager()
login_manager.init_app(app)
Next, define a user loader function, which tells Flask-Login how to load a user from the session. This function should take a user ID and return the corresponding user object:
from your_model import User
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
Now, you can protect routes by requiring users to be logged in. Here’s an example of a login route and a protected dashboard route:
from flask import render_template, redirect, url_for, request, flash
from flask_login import login_user, login_required, logout_user
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
# Logic for authenticating user
user = User.query.filter_by(username=request.form['username']).first()
if user and user.check_password(request.form['password']):
login_user(user)
return redirect(url_for('dashboard'))
else:
flash('Invalid credentials')
return render_template('login.html')
@app.route('/dashboard')
@login_required
def dashboard():
return "Welcome to your dashboard"
@app.route('/logout')
@login_required
def logout():
logout_user()
return redirect(url_for('login'))
In this setup, the login_user()
function logs in the user after
validating their credentials, and the login_required
decorator
ensures that users cannot access certain routes unless they’re logged
in. When the user logs out, logout_user()
clears their session.
Flask-Login handles most of the complexity behind session management and gives you a secure, scalable solution for authentication in Flask apps.
4. Flask-Migrate
Flask-Migrate is an essential extension for any Flask project using Flask-SQLAlchemy. It simplifies the process of managing database migrations using Alembic, a migration tool that allows you to make changes to your database schema without manually writing SQL. With Flask-Migrate, you can effortlessly keep track of your database schema’s evolution, ensuring that updates can be rolled out smoothly in any environment.
Key Features and Strengths
- Allows for smooth database migrations without the need to write raw SQL.
- Integrates seamlessly with Flask-SQLAlchemy, handling schema changes over time.
- Provides command-line tools for generating, upgrading, and downgrading migrations.
Instructions
To get started, first install Flask-Migrate:
pip install flask-migrate
Once Flask-Migrate is installed, you need to configure it in your
application. Start by importing the necessary classes and initializing
the Migrate
object:
from flask_migrate import Migrate
from yourapp import app, db
migrate = Migrate(app, db)
Next, ensure that Flask-SQLAlchemy and Flask-Migrate are properly set
up by initializing them in your app’s entry point (usually app.py
or
__init__.py
). Once the setup is complete, you can start working with
migrations through Flask-Migrate’s command-line interface.
To initialize the migration repository, start by creating a migration folder in your project. You can do this by running the command:
flask db init
After making changes to your database models, you’ll need to generate a migration file. Use the following command to create a new migration:
flask db migrate -m "Add new table or column"
This will create a migration file in the migrations/versions
directory. Once the migration file is generated, you can apply the
migration to your database using:
flask db upgrade
If you ever need to roll back a migration, Flask-Migrate makes that
simple as well. Just run the downgrade
command:
flask db downgrade
By handling these tasks for you, Flask-Migrate ensures that your database schema stays in sync across environments, whether you’re adding new tables, modifying columns, or making larger structural changes.
5. Flask-DebugToolbar
Flask-DebugToolbar is an incredibly useful extension for developers looking to gain deeper insights into their Flask applications during the development process. It provides an interactive debugger and a set of panels displaying detailed information about your application’s requests, SQL queries, templates, and more. With this extension, debugging complex issues and performance bottlenecks becomes much easier, as you can get real-time feedback without slowing down your workflow.
Key Features and Strengths
- Real-time debugging with interactive error pages.
- Panels for inspecting SQL queries, templates, request/response data, and more.
- Displays detailed tracebacks when errors occur, helping to pinpoint issues quickly.
Instructions
To get started with Flask-DebugToolbar, you’ll first need to install it using pip:
pip install flask-debugtoolbar
Once installed, you can enable it in your Flask application by adding it to your app configuration. First, import and initialize the toolbar:
from flask_debugtoolbar import DebugToolbarExtension
app.debug = True # Ensure that Flask is in debug mode
toolbar = DebugToolbarExtension(app)
By default, the toolbar will appear on every page of your app during development. It provides you with interactive panels that display information such as request variables, rendered templates, SQL queries executed during a request, and the time taken for each request.
For additional configuration, such as toggling specific panels on or off, you can add options to your app’s config:
app.config['DEBUG_TB_INTERCEPT_REDIRECTS'] = False
Flask-DebugToolbar is a powerful ally during development, giving you instant access to detailed debugging information. Whether you’re inspecting database queries or checking request headers, it helps streamline the development process by offering real-time insights into your app’s behavior.
6. Flask-Caching
Flask-Caching is a powerful extension that helps improve the performance of your Flask application by reducing the load on your database and other resources. It works by caching data that would otherwise require repeated database queries or expensive computations. By integrating seamlessly with popular caching backends like Redis or Memcached, Flask-Caching allows you to store and quickly retrieve frequently accessed data, speeding up your app considerably.
Key Features and Strengths
- Improves application performance by cutting down on database hits.
- Supports multiple backends, including Redis and Memcached, making it flexible enough to fit various caching needs.
- Simple and straightforward setup and configuration, so you can get up and running quickly.
Instructions
To start using Flask-Caching, install it via pip:
pip install flask-caching
Once installed, you can configure Flask-Caching to work with your desired backend. In this example, we’ll use Redis as the cache store. First, make sure you have Redis running, and then add the necessary configuration in your Flask app:
from flask_caching import Cache
# Define the cache configuration
app.config['CACHE_TYPE'] = 'RedisCache'
app.config['CACHE_REDIS_HOST'] = 'localhost'
app.config['CACHE_REDIS_PORT'] = 6379
# Initialize the cache
cache = Cache(app)
Now, you can use the @cache.cached()
decorator to cache the results
of a specific route. For example, caching the result of a homepage
query:
@app.route('/')
@cache.cached(timeout=60)
def homepage():
# Simulate a heavy query
data = perform_heavy_query()
return render_template('home.html', data=data)
With the example above, the homepage()
view will only execute the
query once every 60 seconds, drastically reducing database
load. Flask-Caching automatically handles storing and retrieving the
cached data from Redis.
Flask-Caching is an easy and effective way to boost the performance of your Flask app, especially for pages or data that don’t need to be recalculated or fetched from the database on every request. With flexible backend support and minimal setup, it’s a great tool for optimizing resource-heavy applications.
7. Flask-Mail
Flask-Mail is an excellent solution for integrating email functionality into your Flask application. Whether you’re building a user registration system, sending password reset links, or setting up notifications, Flask-Mail provides an easy-to-use interface for sending emails through various email services. It works well with popular providers like Gmail, SMTP, and more, and can even send emails asynchronously to improve performance.
Key Features and Strengths
- Simple integration with popular email services like SMTP, Gmail, and others.
- Asynchronous email sending, allowing you to send emails without blocking the main application flow.
- Seamless integration with Flask-WTF forms, making it a perfect companion for user registration and feedback forms.
Instructions
To get started with Flask-Mail, install the extension using pip:
pip install flask-mail
Next, configure Flask-Mail in your application. Add the following configuration for the email server, adjusting the values to suit your email provider (e.g., Gmail or a custom SMTP server):
from flask_mail import Mail, Message
# Configure mail settings
app.config['MAIL_SERVER'] = 'smtp.gmail.com'
app.config['MAIL_PORT'] = 587
app.config['MAIL_USE_TLS'] = True
app.config['MAIL_USERNAME'] = 'your-email@gmail.com'
app.config['MAIL_PASSWORD'] = 'your-email-password'
# Initialize the mail extension
mail = Mail(app)
Once the configuration is complete, you can send emails by creating
and sending a Message
object. Here’s an example of how to send a
test email:
@app.route('/send-email')
def send_email():
msg = Message('Hello from Flask-Mail',
sender='your-email@gmail.com',
recipients=['recipient@example.com'])
msg.body = 'This is a test email sent from a Flask application.'
mail.send(msg)
return 'Email sent!'
To improve performance, you can send emails asynchronously using a background task, ensuring that your main application flow doesn’t get blocked. Here’s a simple example using Python’s threading module:
from threading import Thread
def send_async_email(app, msg):
with app.app_context():
mail.send(msg)
@app.route('/send-email-async')
def send_email_async():
msg = Message('Hello from Flask-Mail',
sender='your-email@gmail.com',
recipients=['recipient@example.com'])
msg.body = 'This is an asynchronous test email sent from Flask.'
Thread(target=send_async_email, args=(app, msg)).start()
return 'Asynchronous email sent!'
Flask-Mail makes it easy to handle email functionality in your Flask app, whether you’re sending transactional emails, notifications, or asynchronous messages. It integrates smoothly into your existing setup and pairs well with Flask-WTF for handling forms like user registration and feedback.
8. Flask-Assets
Flask-Assets is a powerful extension that helps manage static assets like JavaScript, CSS, and image files in Flask applications. It allows you to combine, minify, and bundle these files for better performance and reduced load times. With Flask-Assets, you can also integrate pre-processors such as LESS, SASS, or CoffeeScript, making it easier to organize and optimize your frontend resources.
Key Features and Strengths
- Asset bundling and compression: Automatically combines and minifies your JavaScript and CSS files, reducing the number of HTTP requests and the size of the files being served.
- Supports pre-processors: Works with popular CSS and JavaScript pre-processors like LESS, SASS, and CoffeeScript.
- Simple integration with Flask templates: Easily include asset bundles in your Jinja2 templates for streamlined management of frontend resources.
Instructions
To get started with Flask-Assets, install it using pip:
pip install Flask-Assets
Next, integrate Flask-Assets into your Flask application. First,
import the necessary components and initialize Environment
for asset
management:
from flask_assets import Environment, Bundle
assets = Environment(app)
Now, create asset bundles. For example, to bundle multiple JavaScript and CSS files into single files:
js = Bundle('src/js/main.js', 'src/js/helpers.js', filters='jsmin', output='dist/main.min.js')
css = Bundle('src/css/style.css', 'src/css/theme.css', filters='cssmin', output='dist/style.min.css')
assets.register('js_all', js)
assets.register('css_all', css)
In this setup, the JavaScript and CSS files are being combined and
minified with jsmin
and cssmin
filters, then saved as minified
files in the dist
directory.
To include the bundled assets in your templates, use Jinja2
syntax. For example, in your base.html
template:
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='dist/style.min.css') }}">
</head>
<body>
<script type="text/javascript" src="{{ url_for('static', filename='dist/main.min.js') }}"></script>
</body>
</html>
This will ensure that the bundled and minified assets are used in your app, improving performance by reducing the number of static files served and compressing them for faster load times.
Flask-Assets is a great tool for optimizing the delivery of static files in your Flask applications. By managing your assets through bundles and minification, it ensures your frontend resources are organized and delivered efficiently, contributing to faster page loads and a better user experience.
9. Flask-Dance
Flask-Dance is a useful extension for handling OAuth authentication in Flask applications. It simplifies the integration with third-party providers like Google, GitHub, Twitter, Facebook, and more. Instead of manually handling OAuth flows and writing complex logic for connecting with external services, Flask-Dance provides a clean, straightforward interface to manage these connections securely and efficiently.
Key Features and Strengths
- Pre-built OAuth support for popular services: Integrates with major OAuth providers like Google, GitHub, Facebook, and Twitter out of the box.
- Simple token management: Automatically handles OAuth token storage and retrieval, so you don’t have to manage tokens manually.
- Seamless integration with Flask-Login: Works well with Flask-Login, allowing you to tie OAuth authentication into your existing user management system.
Instructions
First, install Flask-Dance using pip:
pip install Flask-Dance
After installation, configure Flask-Dance for the specific OAuth provider you want to integrate with. In this example, we’ll use Google. Start by importing the necessary components and setting up the Google blueprint:
from flask import Flask, redirect, url_for
from flask_dance.contrib.google import make_google_blueprint
from flask_login import login_user
app = Flask(__name__)
app.secret_key = 'supersecretkey'
google_bp = make_google_blueprint(client_id='your-client-id',
client_secret='your-client-secret',
redirect_to='google_login')
app.register_blueprint(google_bp, url_prefix='/login')
Once configured, create a route to handle the login flow. This route will check if the user is already authorized; if not, it will redirect them to Google’s OAuth flow:
@app.route('/google')
def google_login():
if not google_bp.session.authorized:
return redirect(url_for('google.login'))
resp = google_bp.session.get('/plus/v1/people/me')
assert resp.ok, resp.text
return f"You are {resp.json()['displayName']} on Google"
Flask-Dance automatically handles OAuth token management, so you don’t
need to worry about manually retrieving and storing tokens. The
google_bp.session
object represents an authenticated session with
Google, and you can use it to make API requests on behalf of the
logged-in user.
To integrate OAuth with user sessions, you can tie Flask-Dance with Flask-Login by logging in the user after a successful OAuth flow:
@app.route('/google')
def google_login():
if not google_bp.session.authorized:
return redirect(url_for('google.login'))
# Get user information from Google
resp = google_bp.session.get('/plus/v1/people/me')
assert resp.ok, resp.text
user_info = resp.json()
# Implement custom user login logic here
user = User.query.filter_by(google_id=user_info['id']).first()
if not user:
user = User(google_id=user_info['id'], name=user_info['displayName'])
db.session.add(user)
db.session.commit()
login_user(user)
return redirect(url_for('dashboard'))
In this example, once the user is authenticated through Google, their information is retrieved, and the user is either logged in or added to the database if they don’t already exist. Flask-Dance makes integrating OAuth authentication into your Flask application quick and easy.
Flask-Dance saves time by providing a reliable, secure, and simple way to manage OAuth authentication. Whether you’re allowing users to log in with Google, GitHub, or other providers, this extension handles the complexity of OAuth, letting you focus on building features instead of worrying about authentication flows.
Conclusion
Flask extensions are an essential part of the Flask ecosystem, providing developers with the tools they need to enhance and scale their applications. Whether it’s handling user authentication, managing databases, optimizing performance through caching, or integrating third-party services via OAuth, these extensions add critical functionality without compromising Flask’s lightweight, flexible nature.
By choosing the right extensions—like Flask-SQLAlchemy for database management, Flask-WTF for handling forms, Flask-Login for user authentication, or Flask-Caching for performance optimization—you can build robust, feature-rich web applications quickly and efficiently. These tools help streamline development, reduce repetitive tasks, and provide scalability as your application grows.
No matter your app’s complexity, the right Flask extensions empower you to focus on what matters most: delivering a great product to your users.