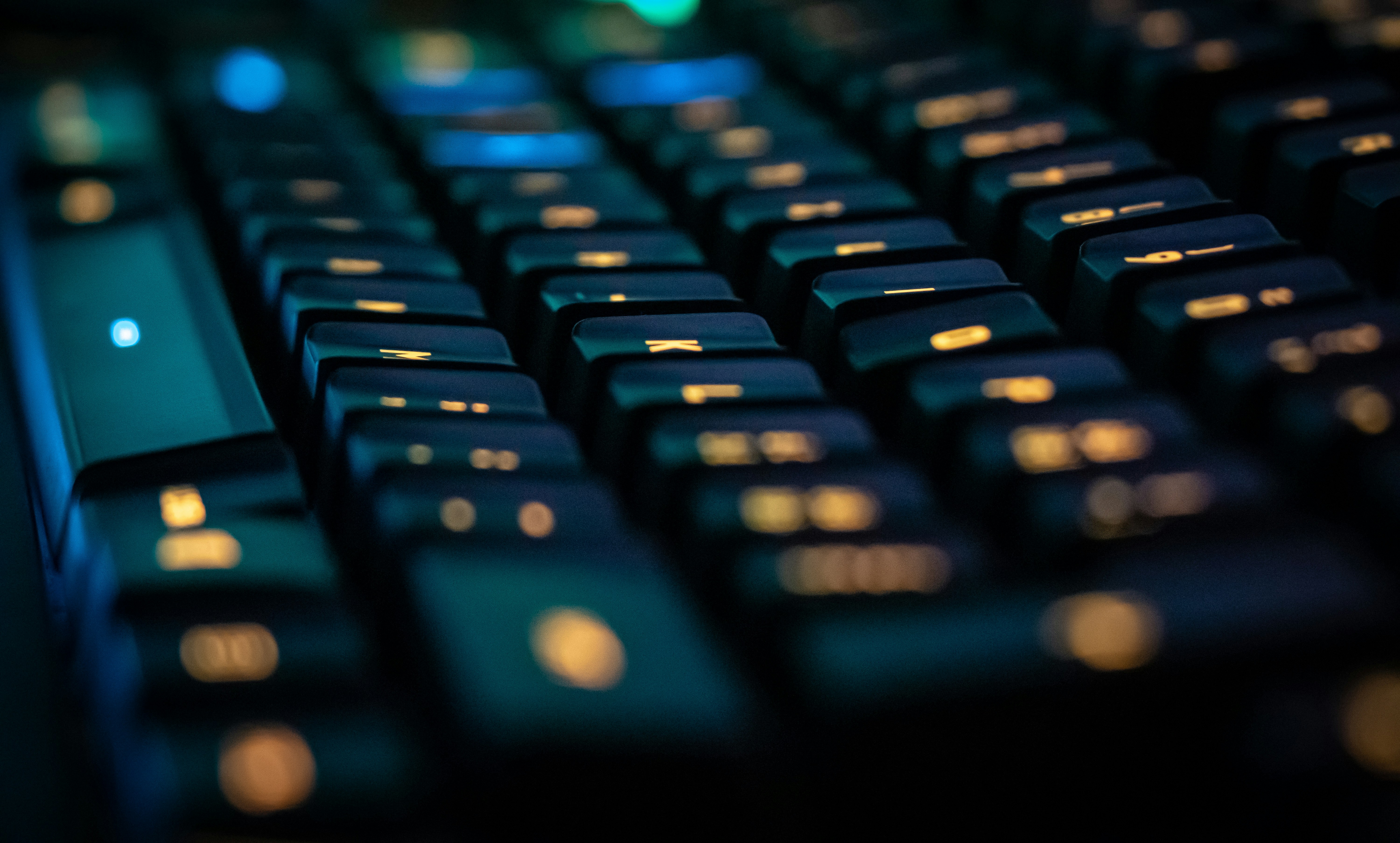
Introduction
Flask, a lightweight and flexible Python web framework, is renowned for its simplicity and power. One of its standout features is the ability to extend functionality through Command Line Interface (CLI) commands, making it easier for developers to automate tasks, manage applications, and interact directly with the app. Flask’s blueprint system further enhances this by allowing you to structure your application into modular components, each with its own set of routes, templates, and commands.
In this tutorial, we’ll walk you through the process of integrating CLI commands within Flask blueprints, enabling you to create custom commands tailored to your application’s specific needs. Whether you’re an experienced Flask developer or just starting out, this guide will give you practical insights into harnessing the full potential of CLI commands to streamline your workflow and increase your app’s flexibility.
Step 1: Setting Up Your Blueprint
Before we begin adding CLI commands, you’ll need to have a blueprint ready. In Flask, a blueprint is a way to organize your application into separate components, each with its own set of routes and templates. If you’re not familiar with blueprints, think of them as mini-applications within your main Flask application.
To create a blueprint, you’ll need to import the Blueprint
class from Flask
and then instantiate it. For instance:
from flask import Blueprint
users_blueprint = Blueprint('users', __name__)
In this example, we’ve created a blueprint named ‘users’. The first argument is
the name of the blueprint which will be used by Flask’s routing mechanism. The
second argument (__name__
) helps Flask locate the blueprint’s resources.
Now that we have our ‘users’ blueprint set up, we can start adding CLI commands to it in the next step.
Step 2: Adding CLI Commands to Your Blueprint
With our blueprint ready, we can now add a custom CLI command. To do this, we’ll
use the cli.command()
decorator provided by Flask. This decorator allows us to
register a function as a command that can be invoked from the command line.
Let’s create a ‘create’ command for our ‘users’ blueprint:
import click
@users_blueprint.cli.command('init_db')
@click.argument('email')
def create(email):
"""Create a new user."""
...
click.echo(f'Added new user (email: {email}!')
In this example, we’ve defined a function named create
and decorated it with
@users_blueprint.cli.command('init_db')
. This means that when you run $ flask
users init_db
, Flask will execute the create
function.
The click.argument('email')
decorator specifies that our command requires one
argument—an email address in this case. Inside the function, we perform some
operations (represented by …) and then use click.echo()
to print out a
success message.
Now you know how to add CLI commands to your Flask blueprints! You can define any number of commands depending on your application’s needs.
Full Working Example
To illustrate a full working example, let’s start with a fresh Python 3 virtual environment with Flask installed:
mkdir cli-test
cd cli-test
python3 -m venv .venv
source .venv/bin/activate
pip3 install flask
Now, create the following app.py
file:
from flask import Flask, Blueprint
import click
# Create a Flask app
app = Flask(__name__)
# Create a default route for demonstration
@app.route('/')
def index():
return "Welcome to the Flask CLI Blueprint Example!"
# Create a blueprint for users
users_blueprint = Blueprint('users', __name__, cli_group='users')
users_blueprint.cli.short_help = "Group of commands for managing users."
# CLI command to initialize the user database
@users_blueprint.cli.command('init_db')
@click.argument('email')
def create(email):
"""Create a new user."""
# Normally, you'd insert the user into a database here
click.echo(f"Added new user with email: {email}")
# Register the blueprint with the Flask app
app.register_blueprint(users_blueprint)
# Ensure the app runs if executed directly
if __name__ == '__main__':
app.run(debug=True)
Now, when we use the flask
command line tool, we can see the new users
command group:
$ flask --help
Usage: flask [OPTIONS] COMMAND [ARGS]...
A general utility script for Flask applications.
Commands:
routes Show the routes for the app.
run Run a development server.
shell Run a shell in the app context.
users Group of commands for managing users.
We provided the cli_group='users'
argument to the Blueprint
to illustrate
how the group name can be customized. It would have defaulted to the name of
the blueprint (also users
in this case).
We can use the --help
argument to show the sub-commands–currently only the
init_db
command we defined:
$ flask users --help
Usage: flask users [OPTIONS] COMMAND [ARGS]...
Options:
--help Show this message and exit.
Commands:
init_db Create a new user.
Notice that the help text for the init_db
subcommand comes from the create
function docstring.
To run the init_db
command:
$ flask users init_db
Usage: flask users init_db [OPTIONS] EMAIL
Try 'flask users init_db --help' for help.
Error: Missing argument 'EMAIL'.
Conveniently, it prompts us to provide the required email address argument.
$ flask users init_db test@example.com
Added new user with email: test@example.com
After using the correct syntax, the command works as expected!
Conclusion
Incorporating CLI commands into Flask blueprints empowers you to take full control of your application’s functionality, providing a direct and efficient way to manage tasks from the command line. By following the steps outlined in this article, you’ve learned how to create and customize commands that can be easily integrated into different parts of your application. Whether you need to manage users, handle database operations, or automate repetitive tasks, CLI commands offer a streamlined solution that enhances your development process.
Mastering this feature not only simplifies complex workflows but also brings scalability and flexibility to your Flask projects. Now, you’re equipped to leverage the power of both Flask’s blueprint architecture and Click’s CLI tools to build more modular, maintainable, and efficient applications.