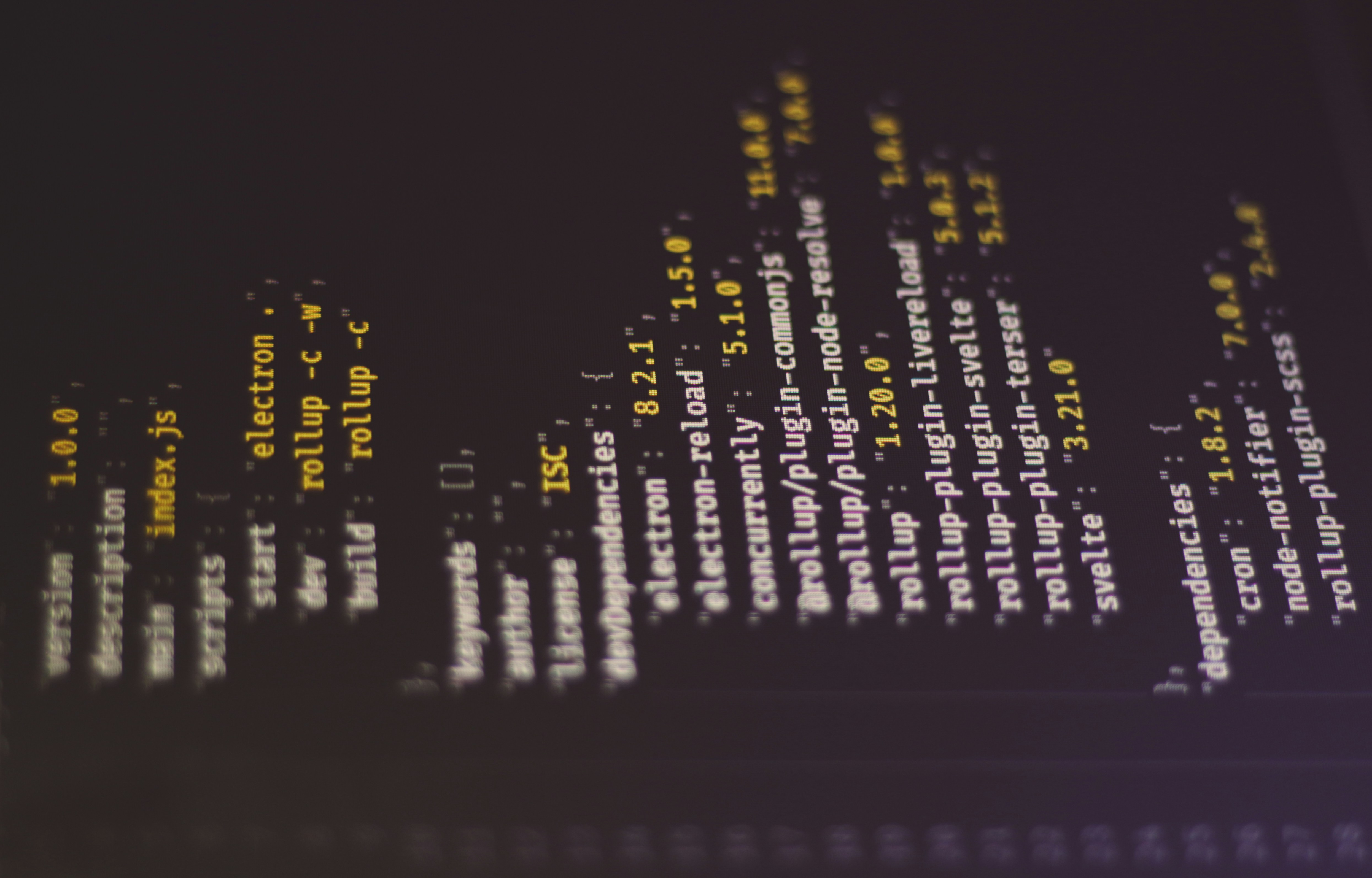
Introduction
Python is a versatile language with many built-in features and packages that make it easy to work with different types of data. One such feature is the ability to pretty print dictionaries, which can be particularly useful when dealing with large datasets or complex nested structures. This article will guide you through the process of pretty printing a dictionary in Python 3 using the json
package. We’ll also briefly mention an alternative method using the pprint
package, but our main focus will be on utilizing JSON for this task.
Step 1: Importing the JSON Package
The first step in pretty printing a dictionary using the json
package is to import it into your Python script. This can be done with a simple import statement at the top of your file. The code snippet below shows how you can do this:
import json
Once you’ve imported the json
package, you’re ready to start working with it. It’s worth noting that if you’re using an Integrated Development Environment (IDE) like PyCharm or Jupyter Notebook, they might automatically import packages for you when they detect their usage in your code.
Step 2: Creating a Dictionary
Before we can pretty print a dictionary, we need to have a dictionary. For the purpose of this guide, let’s create a simple nested dictionary. A nested dictionary is a dictionary within another dictionary. Here’s an example:
my_dict = {
"name": "John Doe", "age": 30,
"children": [ {"name": "Jane Doe", "age": 10}, {"name": "Jim Doe", "age": 7} ]
}
In this example, my_dict
is a Python dictionary that contains information about John Doe and his children. We’ll use this as our data for pretty printing.
Step 3: Pretty Printing the Dictionary
Now that we have our dictionary and the json
package imported, we can pretty print our dictionary. The json
package provides a method called dumps()
which can be used to format a Python object into a JSON string. It takes two arguments: the data you want to convert and an optional parameter called indent
, which specifies how many spaces to use for indentation.
Here’s how you can use it:
print(json.dumps(my_dict, indent=4))
When you run this code, it will print out your dictionary in a nicely formatted way with 4 spaces of indentation:
{
"name": "John Doe",
"age": 30,
"children": [
{
"name": "Jane Doe",
"age": 10
},
{
"name": "Jim Doe",
"age": 7
}
]
}
Step 4: Alternative Method Using pprint
While our focus is on the json
package, it’s worth mentioning that Python also has a built-in module for pretty printing called pprint
. This can be used as an alternative to the json
package. To use it, you would import the pprint
module and then call its pprint()
function with your dictionary as an argument.
Here’s how you can do this:
import pprint
pp = pprint.PrettyPrinter(indent=4)
pp.pprint(my_dict)
This will produce the following output:
{ 'age': 30,
'children': [ {'age': 10, 'name': 'Jane Doe'},
{'age': 7, 'name': 'Jim Doe'}],
'name': 'John Doe'}
Conclusion
Pretty printing dictionaries in Python can greatly enhance readability, especially when dealing with large or nested data structures. While there are several ways to achieve this, using the json
package provides a straightforward and effective method. The pprint
module is another viable option that comes built-in with Python. Regardless of the method you choose, being able to clearly visualize your data structures will undoubtedly aid in your programming tasks.